C# 文字列を日付型に変換する
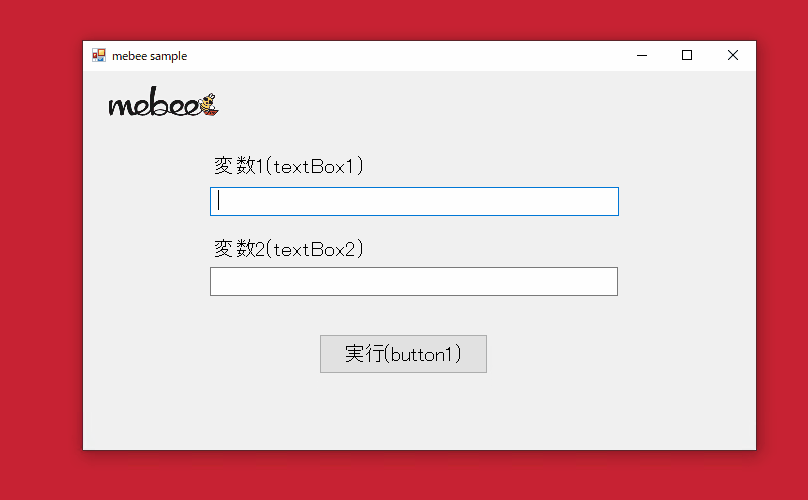
C#で、DateTime.ParseExactメソッドを使用して、文字列を日付型に変換するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
DateTime.ParseExact使い方
DateTime.ParseExactを使用すると、文字列を日付型に変換することが可能です。
// 書式を設定して日付型に変換
DateTime dt = DateTime.ParseExact("20201201", "yyyyMMdd", null);
// 表示
Console.WriteLine(dt.ToString()); // 2020/12/01 0:00:00
// yyyymmddhhmmss 形式を設定して日付型に変換
dt = DateTime.ParseExact("20201211231254", "yyyyMMddHHmmss", null);
// 表示
Console.WriteLine(dt.ToString()); // 2020/12/11 23:12:54
// yyyy年MM月dd日 HH時mm分ss秒 形式を設定して日付型に変換
dt = DateTime.ParseExact("2020年12月25日 12時34分56秒", "yyyy年MM月dd日 HH時mm分ss秒", null);
// 表示
Console.WriteLine(dt.ToString()); // 2020/12/25 12:34:56
サンプルコード
以下は、
実行ボタンをクリックすると、textboxに入力した文字列を別のテキストフォームに日付型に変更して表示する
サンプルコードとなります。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace FormTestApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
DateTime x;
x = DateTime.ParseExact(textBox1.Text, "yyyyMMdd", null); // 変数1
textBox2.Text = x.ToString();
}
}
}
日付型に変更されて表示されることが確認できます。
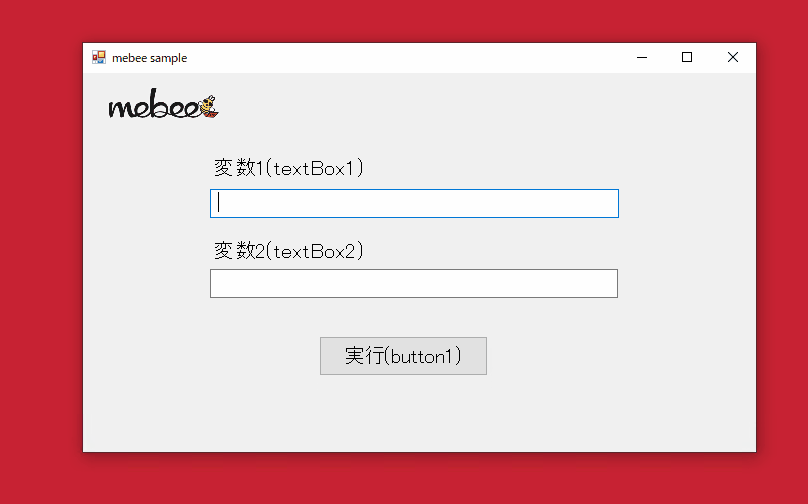
-
前の記事
javascript imgタグの画像をbase64文字列に変換する 2020.10.09
-
次の記事
javascript 指定したクラスを使用しているかを確認する 2020.10.10
コメントを書く