C# ランダムな文字列を生成する
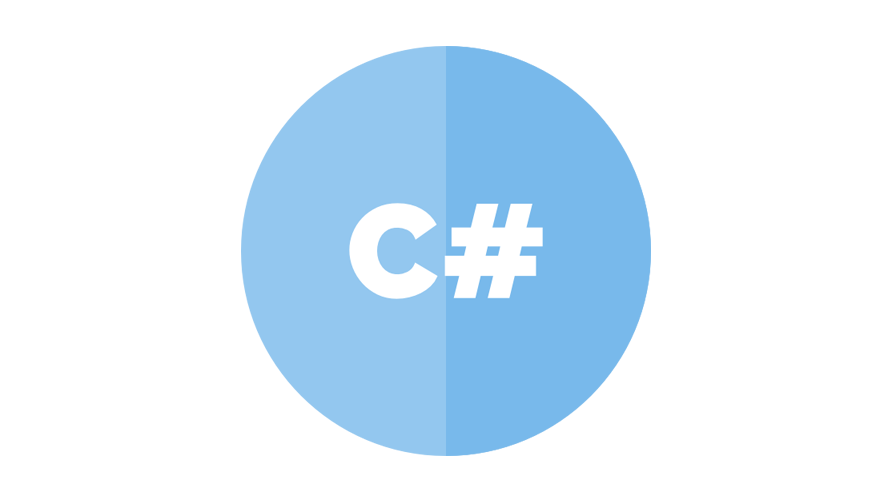
C#で、ランダムな文字列を生成するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
ランダムな文字列を生成する
ランダムな文字列を生成するには、
予め必要な文字列を用意して、ランダムな位置を乱数で指定して文字列に結合していきます。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
// 文字列を用意
var txt = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
var n = 10; // 10文字生成
// 乱数発生用
var random = new Random();
string result = "";
for (int i = 0; i < n; i++)
{
result += txt[random.Next(txt.Length)]; // 文字数の範囲内で乱数を生成
}
Console.WriteLine(result);
}
}
}
実行結果

漢字やひらがなも用意すれば使用できます。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
// 文字列を用意
var txt = "あいうえお漢字";
var n = 10; // 10文字生成
// 乱数発生用
var random = new Random();
string result = "";
for (int i = 0; i < n; i++)
{
result += txt[random.Next(txt.Length)]; // 文字数の範囲内で乱数を生成
}
Console.WriteLine(result);
}
}
}
実行結果
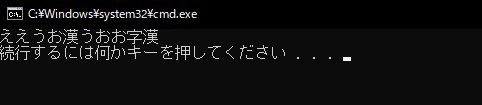
Linq
「Linq」を使用する方法もあります。
using System;
using System.Linq;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
// 文字列を用意
var txt = "あいうえお漢字";
var n = 10; // 10文字生成
// 乱数発生用
var random = new Random();
string result = "";
result = new string(Enumerable.Repeat(txt, n)
.Select(x => x[random.Next(x.Length)]).ToArray());
Console.WriteLine(result);
}
}
}
-
前の記事
Oracle Database マテリアライズドビューの指定した更新時間を変更する 2022.07.09
-
次の記事
sqlite 列の合計値を取得する 2022.07.10
コメントを書く