C# 文字列の先頭の文字だけを大文字に変換する
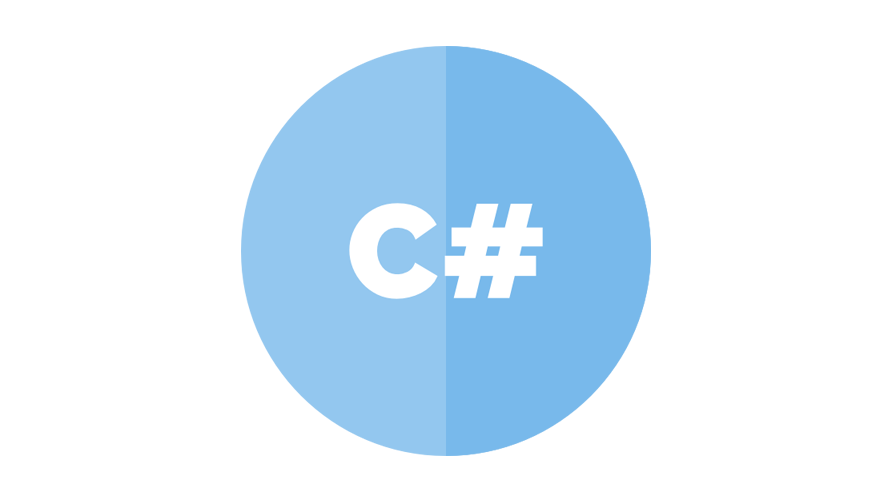
C#で、文字列の先頭の文字だけを大文字に変換するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
文字列の先頭の文字だけを大文字に変換
文字列の先頭の文字だけを大文字に変換するには、
文字列を配列として取得して先頭だけを「ToUpper」で大文字に変換して残った文字と結合します。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "mebee";
Console.WriteLine( char.ToUpper(txt[0]) + txt.Substring(1) );
}
}
}
実行結果

全角文字にも使用することも可能です。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "mebee";
Console.WriteLine( char.ToUpper(txt[0]) + txt.Substring(1) );
Console.WriteLine( Regex.Replace(txt, "^[a-z]", x => x.Value.ToUpper()) );
// 配列にしてから実行
char[] ch = txt.ToCharArray();
ch[0] = char.ToUpper(ch[0]);
Console.WriteLine(new string(ch));
}
}
}
実行結果

ToCharArray
「ToCharArray」を使用して、配列に変換してから大文字に変更する方法もあります。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "mebee";
// 配列にしてから実行
char[] ch = txt.ToCharArray();
ch[0] = char.ToUpper(ch[0]);
Console.WriteLine(new string(ch));
}
}
}
Regex.Replace
正規表現を使用して、先頭だけ大文字にすることも可能です。
using System;
using System.Text.RegularExpressions;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "mebee";
Console.WriteLine( Regex.Replace(txt, "^[a-za-z]", x => x.Value.ToUpper()) );
}
}
}
-
前の記事
kotlin エラー「error: cannot create an instance of an abstract class」の解決方法 2022.07.01
-
次の記事
javascript うるう年の判定処理で「if文」と「new Date」とのパフォーマンスを計測する 2022.07.02
コメントを書く