C# IEnumerableから値を取得する
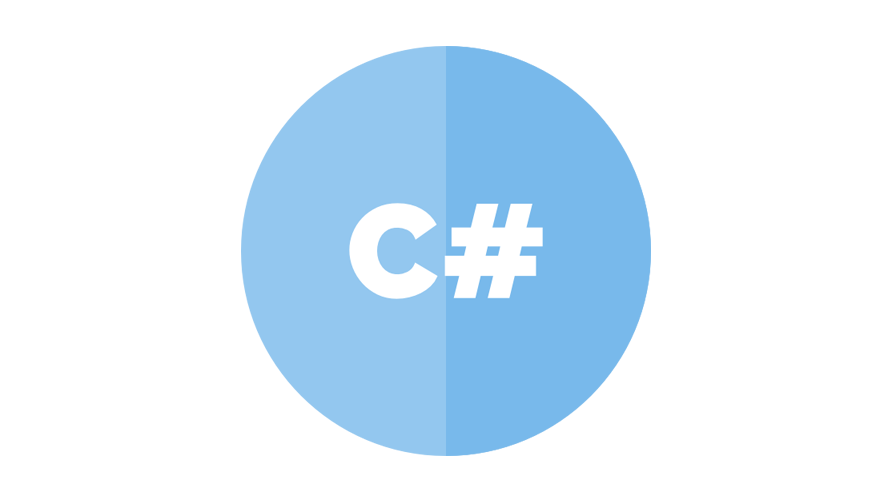
C#で、IEnumerableから値を取得するサンプルコードを記述してます。「foreach」や「Linq」を使用することで取得することが可能です。
環境
- OS windows11 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.4.0
IEnumerable使い方
IEnumerableを使用すると、要素を1つずつ取得することが可能です。
IEnumerable<int> num = new[] { 1, 2, 3, 4, 5 };
以下は、「IEnumerable」の値を取得して表示するサンプルコードとなります。
ソースコード
using System;
using System.Collections.Generic;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
IEnumerable<int> num = new[] { 1, 2, 3, 4, 5 };
foreach (var item in num)
{
Console.Write(item + ", "); // 1, 2, 3, 4, 5
}
Console.ReadKey();
}
}
}
実行結果

「forEach」ではなく「Linq」を使用することも可能です。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
IEnumerable<int> num = new[] { 1, 2, 3, 4, 5 };
Console.WriteLine(String.Join(", ", num.Select(i => i)));
Console.ReadKey();
}
}
}
-
前の記事
AlmaLinux 画像編集ソフトInkscapeをインストールする 2022.01.03
-
次の記事
javascript lodashを使ってオブジェクトのパスを指定してメソッドを実行する 2022.01.04
コメントを書く