C# 配列またはリストの数をカウントする
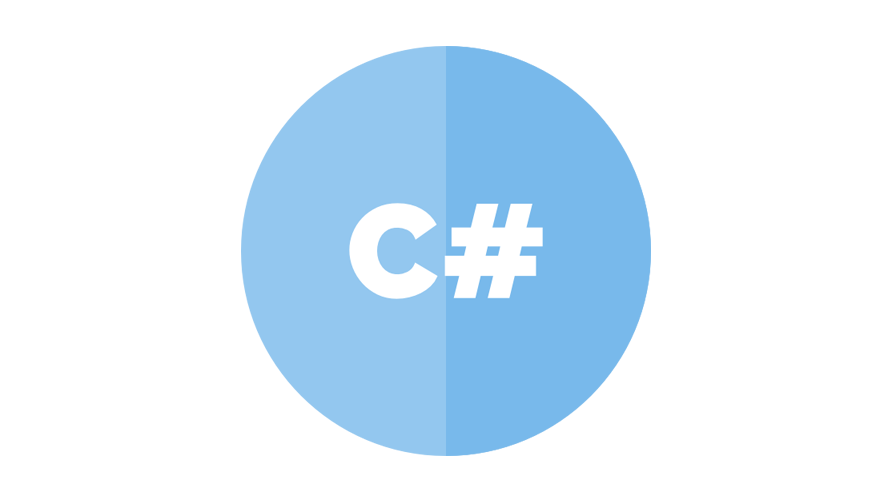
C#で配列またはリストの数をカウントするサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
数をカウント
数をカウントするには、Linqの「Count」を使用することで可能です。
// 配列を用意
int[] num = new int[] { 1, 2, 3, 4, 5 };
// 要素の数をカウント
num.Count(); // 5
以下は、要素の数をカウントして、結果を表示するだけのコードとなります。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
int[] arr = new int[] { 0, 1, 2, 3, 4, 5, 1, 2};
List<int> list = new List<int> { 0, 1, 2, 1, 2 };
try
{
int reault1 = arr.Count();
int reault2 = list.Count();
Console.WriteLine($"実行結果は{reault1}です"); // 8
Console.WriteLine($"実行結果は{reault2}です"); // 5
}
catch (Exception e)
{
System.Console.WriteLine(e.ToString());
}
}
}
}
実行結果

以下のように条件を指定することも可能です。
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
int[] arr = new int[] { 0, 1, 2, 3, 4, 5, 1, 2};
List<int> list = new List<int> { 0, 1, 2, 1, 2 };
try
{
int reault1 = arr.Count(v => v % 2 == 0);
int reault2 = list.Count(v => v > 1 );
Console.WriteLine($"実行結果は{reault1}です"); // 4
Console.WriteLine($"実行結果は{reault2}です"); // 2
}
catch (Exception e)
{
System.Console.WriteLine(e.ToString());
}
}
}
}
実行結果
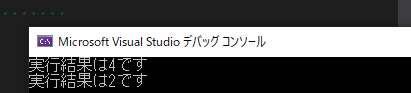
-
前の記事
MySQL 現在のパケットの最大値を取得する 2022.03.03
-
次の記事
javascript エラー「SyntaxError: Unexpected EOF」の解決方法 2022.03.03
コメントを書く