node.js PostgreSQLに接続する
- 作成日 2021.10.22
- node.js PostgreSQL
- node.js, PostgreSQL
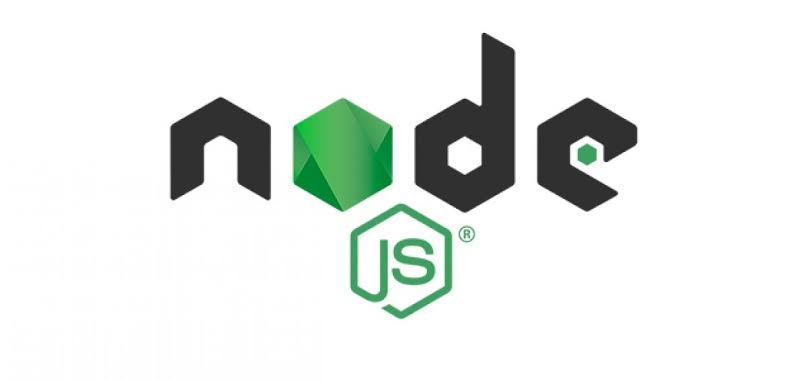
node.jsのライブラリ「pg」でPostgreSQLに接続するサンプルコードを記述してます。nodeのバージョンは14.15.1となります。
環境
- OS Ubuntu 20.10
- node V14.15.1
- npm 6.14.9
- PostgreSQL 13.1
pgインストール
pgを使用して、接続するので、npmでインストールしておきます。
npm i pg
データベース接続
「sample」というデータベースに接続して、バージョン情報を取得するだけのサンプルコードとなります。
const { Client } = require('pg')
const pg = new Client({
user: 'mebee',
host: '0.0.0.0',
database: 'sample',
password: 'password',
port: 5432,
})
pg.connect()
pg.query('SELECT version()', (err, result) => {
if (err) throw err;
console.log(result.rows[0])
pg.end()
})
実行結果
Result {
command: 'SELECT',
rowCount: 1,
oid: null,
rows: [
{
version: 'PostgreSQL 13.1 (Debian 13.1-1.pgdg100+1) on x86_64-pc-linux-gnu, compiled by gcc (Debian 8.3.0-6) 8.3.0, 64-bit'
}
],
fields: [
Field {
name: 'version',
tableID: 0,
columnID: 0,
dataTypeID: 25,
dataTypeSize: -1,
dataTypeModifier: -1,
format: 'text'
}
],
_parsers: [ [Function: noParse] ],
_types: TypeOverrides {
_types: {
getTypeParser: [Function: getTypeParser],
setTypeParser: [Function: setTypeParser],
arrayParser: [Object],
builtins: [Object]
},
text: {},
binary: {}
},
RowCtor: null,
rowAsArray: false
}
実行結果が全て取得されているので、バージョン情報だけを取得したい場合は、以下のようにします。
console.log(result.rows[0])
<出力結果>
{
version: 'PostgreSQL 13.1 (Debian 13.1-1.pgdg100+1) on x86_64-pc-linux-gnu, compiled by gcc (Debian 8.3.0-6) 8.3.0, 64-bit'
}
また、thenやcatchを使用して、以下のように記述することも可能です。
const { Client } = require('pg')
const pg = new Client({
user: 'mebee',
host: '0.0.0.0',
database: 'sample',
password: 'password',
port: 5432,
})
pg.connect()
.then(() => console.log("接続完了"))
.then(() => pg.query("SELECT version()"))
.then(result => console.log(result.rows[0]))
.catch((err => console.log(err)))
.finally((() => pg.end()))
-
前の記事
コマンドプロンプト 入力している状態を一括クリアする 2021.10.22
-
次の記事
netstatを同一ネットワークの別マシンで実行する 2021.10.22
コメントを書く