node.js PostgreSQLにinsertを行う
- 作成日 2021.09.19
- node.js PostgreSQL
- node.js
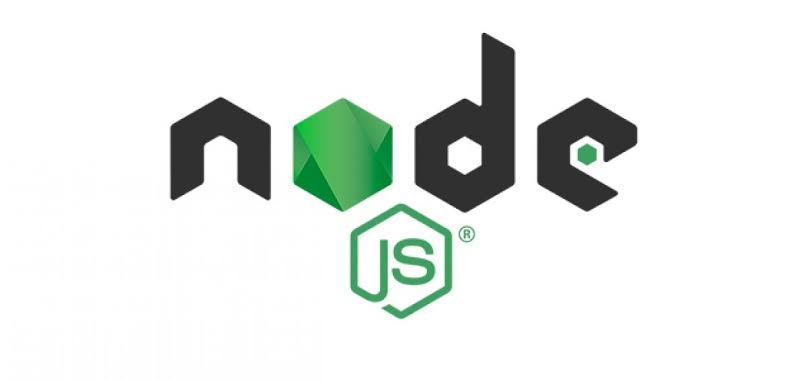
node.jsのライブラリ「pg」でPostgreSQLにinsertを行うサンプルコードを記述してます。nodeのバージョンは14.15.1となります。
環境
- OS Ubuntu 20.10
- node V14.15.1
- npm 6.14.9
- PostgreSQL 13.1
pgインストール
pgを使用して、接続するので、npmでインストールしておきます。
npm i pg
insert実行
事前に「numbers」というテーブルを「sample」データベースに作成しておきます。
create table numbers (
id integer NOT NULL,
name varchar(10),
PRIMARY KEY (id)
);
以下は、「sample」というデータベースに接続して、「numbers」というテーブルにinsertを実行するサンプルコードとなります。
const { Client } = require('pg')
const pg = new Client({
user: 'mebee',
host: '0.0.0.0',
database: 'sample',
password: 'password',
port: 5432,
})
const sql = "INSERT INTO numbers (id, name) VALUES ($1, $2)"
const values = [1, 'mebee']
pg.connect()
.then(() => console.log("接続完了"))
.then(() => pg.query(sql, values))
.then(result => console.log(result))
.catch((err => console.log(err)))
.finally((() => pg.end()))
実行結果
接続完了
Result {
command: 'INSERT',
rowCount: 1,
oid: 0,
rows: [],
fields: [],
_parsers: undefined,
_types: TypeOverrides {
_types: {
getTypeParser: [Function: getTypeParser],
setTypeParser: [Function: setTypeParser],
arrayParser: [Object],
builtins: [Object]
},
text: {},
binary: {}
},
RowCtor: null,
rowAsArray: false
}
データが挿入されていることが確認できます。
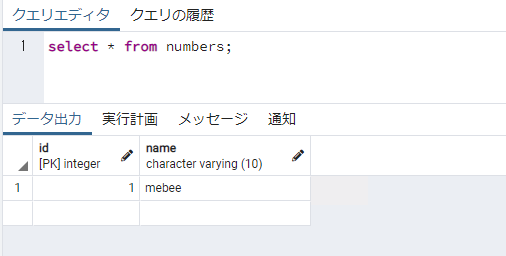
-
前の記事
Linux moreコマンド実行中にファイルの行数を表示する 2021.09.19
-
次の記事
javascript イベント発生からイベントバブル(親要素までイベントが発生するか)を判定する 2021.09.20
コメントを書く