java 文字列が同じであるかを判定する
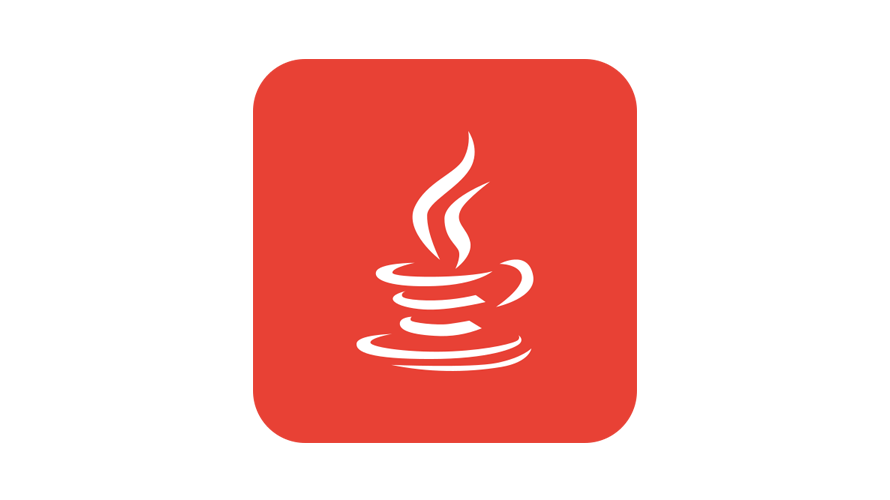
javaで、文字列が同じであるかを判定する手順を記述してます。「equals」に文字列を指定して判定します。
環境
- OS windows11 home
- java 19.0.1
手順
文字列が同じであるかを判定するには、「equals」を使用することで可能です。
文字列.equals(文字列)
実際に、使用してみます。
public class App {
public static void main(String[] args) throws Exception {
String str1 = "mebee";
String str2 = "mebee";
String str3 = "MEBEE";
System.out.println(str1.equals(str2)); // true
System.out.println(str1.equals(str3)); // false
}
}
判定されていることが確認できます。
「null」を使用すると「NullPointerException」が発生します。
public class App {
public static void main(String[] args) throws Exception {
String str1 = null;
String str2 = "mebee";
System.out.println(str1.equals(str2))
// Exception in thread "main" java.lang.NullPointerException:
// Cannot invoke "String.equals(Object)" because "<local1>" is null
// at App.main(App.java:7)
}
}
「==」を使用すれば「null」同士の比較は可能です。
public class App {
public static void main(String[] args) throws Exception {
String str1 = null;
String str2 = null;
String str3 = "mebee";
System.out.println(str1 == str2); // true
System.out.println(str1 == str3); // false
}
}
-
前の記事
GAS スプレッドシートの行の前後を指定して列を追加する 2024.03.14
-
次の記事
PyCharm 置換を開くショートカットキー 2024.03.15
コメントを書く