kotlin 抽象クラスを作成する
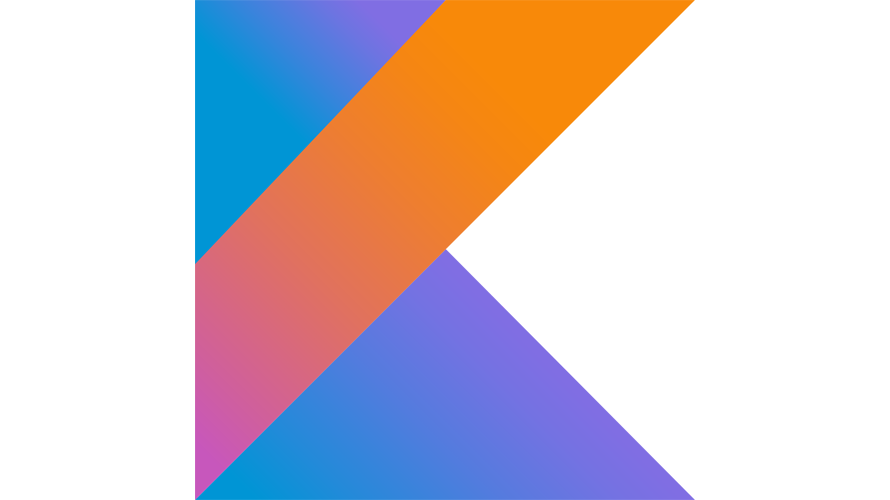
kotlinで、クラスを継承する手順を記述してます。「abstract」を使用します。
環境
- OS windows11 home
- java 19.0.1
- kotlin 1.7.20-release-201
手順
抽象クラスするには、以下の構文で可能です。「abstract」で指定したメソッドは必ずオーバーライドする必要があります。
// 抽象クラス
abstract class クラス名{
abstract fun メソッド名
}
// サブクラス
class クラス名() : 抽象クラス名() {
override fun メソッド名
}
実際に、抽象クラスを作成してオーバーライドしてみます。
abstract class Hoge {
abstract fun f()
}
class Foo() : Hoge() {
override fun f() { println("Foo") }
}
fun main(){
val bar = Foo()
bar.f() // Foo
}
オーバーライドされていることが確認できます。
抽象クラスはオーバーライドせずに使用するとエラーとなります。
abstract class Hoge {
abstract fun f()
}
class Foo() : Hoge() {
override fun f() { println("Foo") }
}
fun main(){
val hoge = Hoge() // error: cannot create an instance of an abstract class
hoge.f()
val foo = Foo()
foo.f()
}
また、抽象クラスで指定しているメソッドをオーバーライドしないとエラーになります。
abstract class Hoge {
abstract fun f()
abstract fun g()
}
class Foo() : Hoge() {
// error: class 'Foo' is not abstract and does not implement abstract base
// class member public abstract fun g(): Unit defined in Hoge
override fun f() { println("Foo") }
}
fun main(){
val foo = Foo()
foo.f()
}
-
前の記事
java ファイルが読み取り可能であるかを判定する 2023.04.04
-
次の記事
java 絶対値を求める 2023.04.05
コメントを書く