C# 文字列が正の整数であるかを判定する
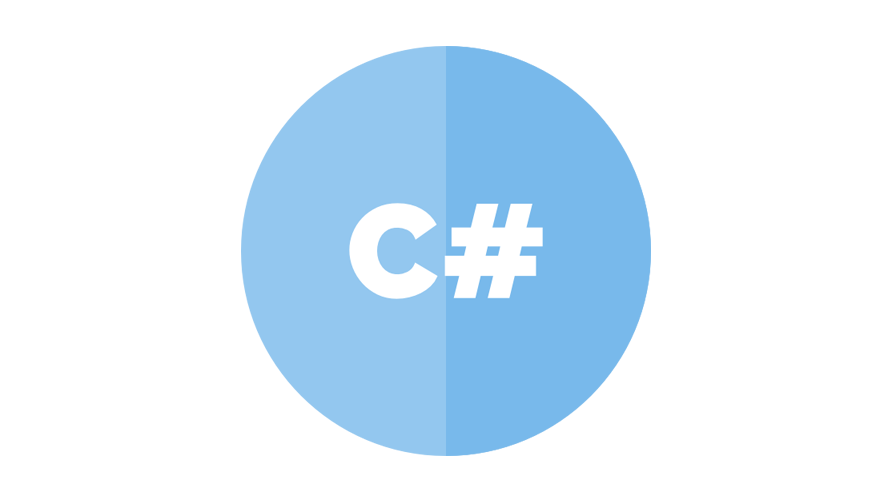
C#で、文字列が正の整数であるかを判定するサンプルコードを記述してます。「Regex.IsMatch」で正規表現を使用することで可能です。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2022 Version 17.2.3
文字列が正の整数であるかを判定
文字列が正の整数であるかを判定するには、「Regex.IsMatch」で正規表現を使用します。
using System;
using System.Text.RegularExpressions;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "123";
if (Regex.IsMatch(txt, @"^[0-9]+$"))
{
Console.WriteLine("数値です");
}
else
{
Console.WriteLine("数値ではありません");
}
}
}
}
実行結果をみると、判定されていることが確認できます。

関数化して、他のパターンを判定してみます。
using System;
using System.Text.RegularExpressions;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "123";
Console.WriteLine(Chk(txt)); // true
txt = "012";
Console.WriteLine(Chk(txt)); // true
txt = "123";
Console.WriteLine(Chk(txt)); // false
txt = "A";
Console.WriteLine(Chk(txt)); // false
}
static Boolean Chk(String txt)
{
return Regex.IsMatch(txt, @"^[0-9]+$");
}
}
}
判定されていることが確認できます。
全角の数値も判定するのであれば、正規表現部分を以下のように変更します。
Regex.IsMatch(txt, @"^[\d]+$");
char.IsDigit
「char.IsDigit」を使用しても、判定することは可能です。
using System;
using System.Linq;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "123";
Console.WriteLine(Chk(txt)); // true
txt = "012";
Console.WriteLine(Chk(txt)); // true
txt = "123";
Console.WriteLine(Chk(txt)); // true
txt = "A";
Console.WriteLine(Chk(txt)); // false
}
static Boolean Chk(String txt)
{
return txt.All(char.IsDigit);
}
}
}
Int32.TryParse
「Int32.TryParse」を使用することもできます。全角は数値として判定されません。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "123";
Console.WriteLine(Chk(txt)); // true
txt = "012";
Console.WriteLine(Chk(txt)); // true
txt = "123";
Console.WriteLine(Chk(txt)); // false
txt = "A";
Console.WriteLine(Chk(txt)); // false
}
static Boolean Chk(String txt)
{
int n;
return Int32.TryParse(txt, out n);
}
}
}
foreach
「foreach」で1つずつ判定する方法もあります。パフォーマンス的にいえば、こちらが一番よさそうです。
using System;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string txt = "123";
Console.WriteLine(Chk(txt)); // true
txt = "012";
Console.WriteLine(Chk(txt)); // true
txt = "123";
Console.WriteLine(Chk(txt)); // false
txt = "A";
Console.WriteLine(Chk(txt)); // false
}
static Boolean Chk(String txt)
{
foreach (char c in txt)
{
if (c >= '0' && c <= '9')
{
return true;
}
}
return false;
}
}
}
-
前の記事
safari リーダーを開くショートカットキー 2023.03.03
-
次の記事
python 文字列から半角空白を除去する 2023.03.03
コメントを書く