Ruby mongoDBに接続してドキュメントを追加する
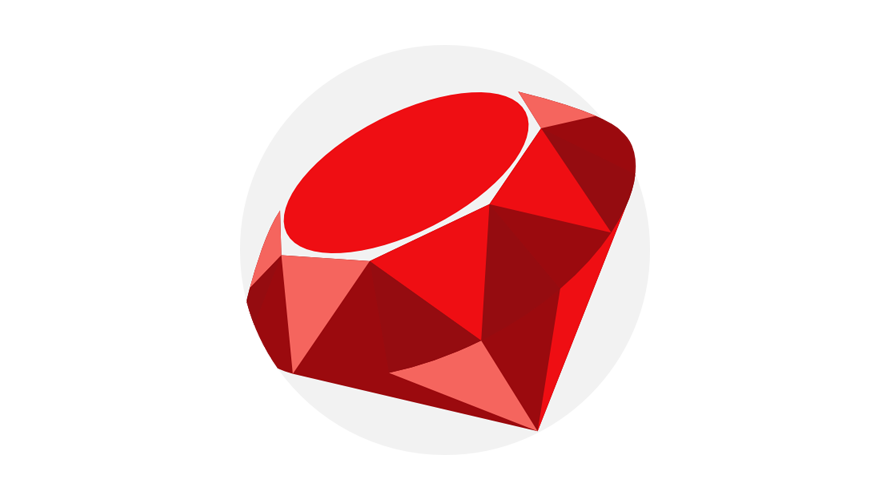
Rubyで、mongoDBに接続してドキュメントを追加するソースコードを記述してます。ライブラリ「mongo」を使用してます。
環境
- OS windows11 home
- ruby ruby 3.0.3p157 (2021-11-24 revision 3fb7d2cadc) [x64-mingw32]
- mongoDB 5.2.0
- vscode 1.63.2
mongoインストール
ライブラリ「mongo」を使います。ない場合は「gem」を使ってインストールしておきます。
gem install mongo
サンプルコード
localhostにある「mongoDB」に接続して、以下のDB「hoge」にあるコレクション「foo」のドキュメントを使用して、サンプルコードを実行してみます。
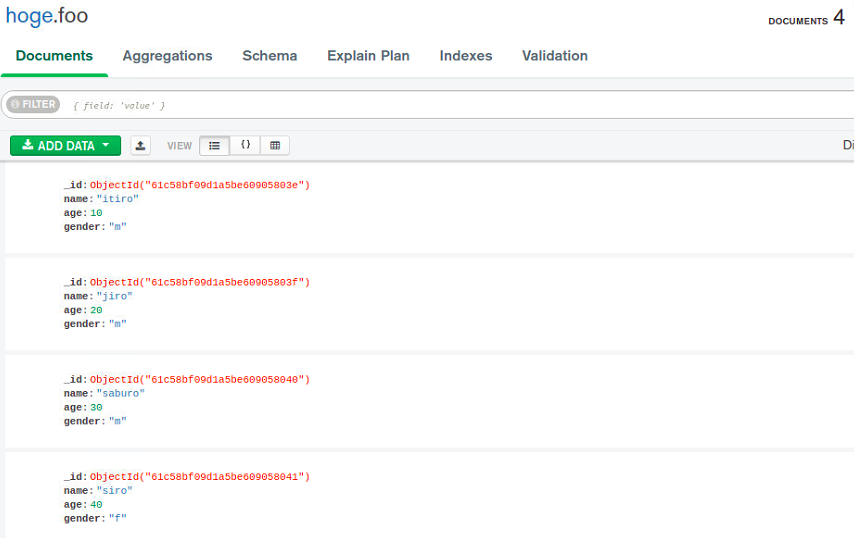
ここでは「test.rb」という名前で作成してます。1件だけ追加するコードを作成してみます。
require "mongo"
Mongo::Logger.logger.level = ::Logger::FATAL
begin
c = Mongo::Client.new(["127.0.0.1:27017"])
d = c.use("hoge")
data = { :_id => BSON::ObjectId.new, :name => "goro", :age => 50, :gender => "x" }
d[:foo].insert_one data
rescue Mongo::Error::NoServerAvailable => e
p e
end
実行結果を確認すると、指定したデータが追加されていることが確認できます。
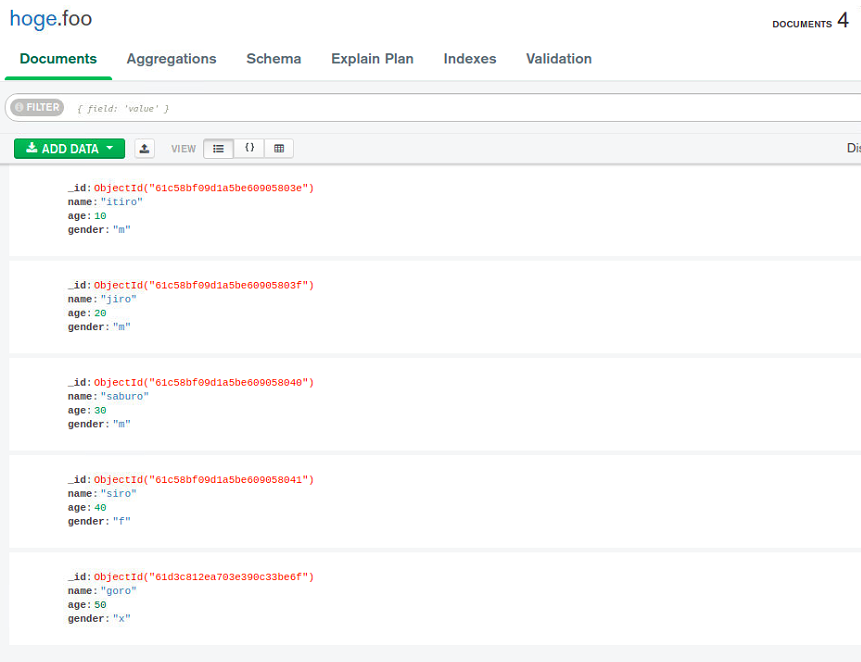
複数追加
複数の値を追加するには「insert_many」を使用します。
require "mongo"
Mongo::Logger.logger.level = ::Logger::FATAL
begin
c = Mongo::Client.new(["127.0.0.1:27017"])
d = c.use("hoge")
d[:foo].insert_many([
{ :_id => BSON::ObjectId.new, :name => "rokuro", :age => 60, :gender => "x" },
{ :_id => BSON::ObjectId.new, :name => "nanaro", :age => 70, :gender => "f" }
])
rescue Mongo::Error::NoServerAvailable => e
p e
end
実行結果
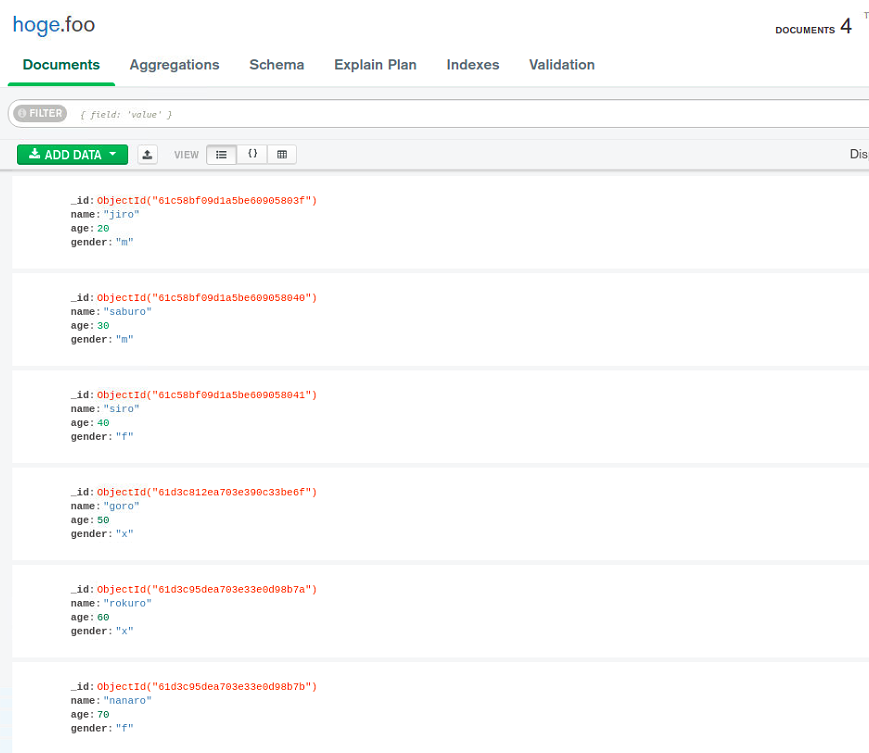
変数を使用することで、結果を取得することも可能です。
require "mongo"
Mongo::Logger.logger.level = ::Logger::FATAL
begin
c = Mongo::Client.new(["127.0.0.1:27017"])
d = c.use("hoge")
result = d[:foo].insert_many([
{ :_id => BSON::ObjectId.new, :name => "rokuro", :age => 60, :gender => "x" },
{ :_id => BSON::ObjectId.new, :name => "nanaro", :age => 70, :gender => "f" }
])
p result
rescue Mongo::Error::NoServerAvailable => e
p e
end
実行結果
> ruby test.rb
#<Mongo::BulkWrite::Result:0x000001705fd6e540 @results={"n_inserted"=>2, "n"=>2, "inserted_ids"=>[BSON::ObjectId('61d3ca09ea703e37e87861a4'), BSON::ObjectId('61d3ca09ea703e37e87861a5')]}>
-
前の記事
sakuraエディタ 現在の開いてるファイル名をコピーするショートカットキー 2022.12.15
-
次の記事
mongoDB 先頭にあるドキュメント(レコード)だけを削除する 2022.12.15
コメントを書く