javascript エラー「SyntaxError: JSON Parse error: Unexpected identifier “object”」の解決方法
- 作成日 2022.07.16
- javascript
- javascript
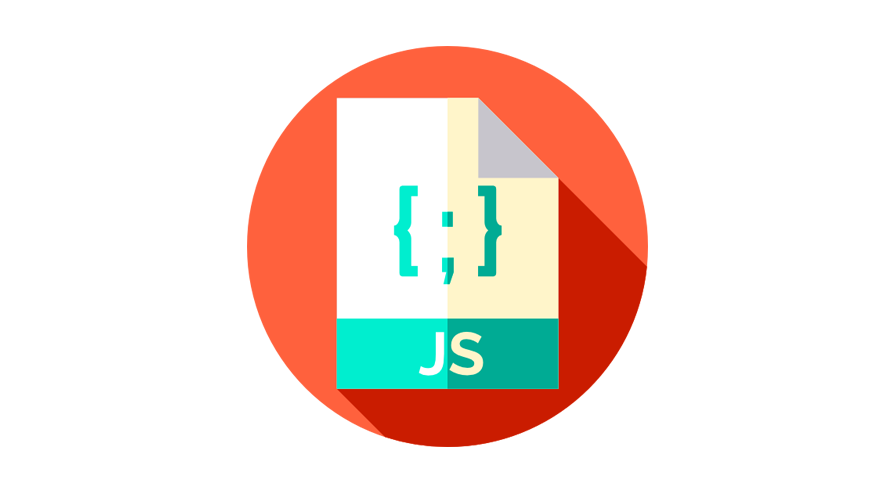
JSON.parse実行時に、エラー「SyntaxError: JSON Parse error: Unexpected identifier “object”」の解決方法を記述してます。
環境
- OS macOS Big Sur
- ブラウザ safari 15.5
エラー内容
以下のコードを実行時に発生
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
async function foo() {
try {
const result = await axios.get('https://jsonplaceholder.typicode.com/todos/1');
console.log( JSON.parse(result.data) );
} catch (err) {
console.error(err);
}
}
foo()
</script>
jsonデータ

エラーメッセージ(safari 15.5)
SyntaxError: Unexpected token o in JSON at position 1
at JSON.parse (<anonymous>)
at jsonGet
原因
文字列が「” (ダブルクォート)」で囲まれていないため発生してます。
const json = '{ a : 100, b : 200, c : 300 }';
const obj = JSON.parse(json);
// SyntaxError: JSON Parse error: Expected '}'
ダブルクォートで囲むとエラーにはなりません。
const json = '{ "a" : 100, "b" : 200, "c" : 300 }';
const obj = JSON.parse(json);
解決方法
「JSON.stringify」で文字列化してから「JSON.parse」を実行する
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script>
async function foo() {
try {
const result = await axios.get('https://jsonplaceholder.typicode.com/todos/1');
console.log( JSON.parse(JSON.stringify(result.data)) );
} catch (err) {
console.error(err);
}
}
foo()
// {userId: 1, id: 1, title: 'delectus aut autem', completed: false}
</script>
JSON.stringify後の「json」は以下となります。

-
前の記事
javascript videoタグの再生速度変更時のイベントを取得する 2022.07.15
-
次の記事
PyCharm コメントアウトを実行するショートカットキー 2022.07.16
コメントを書く