C# 配列またはリストのインデックス番号を指定して値を取得する
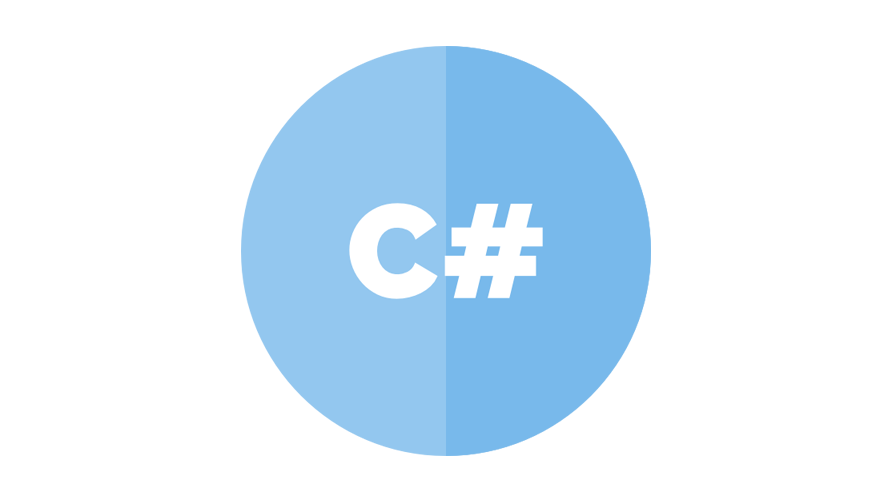
C#で、配列またはリストのインデックス番号を指定して値を取得するサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
インデックス番号を指定して値を取得
インデックス番号を指定して値を取得するには、Linqの「ElementAt」を使用することで可能です。
// 配列を用意
int[] num = new int[] { 1, 2, 3 };
// INDEX番号を指定
num.ElementAt(1); // 2
以下は、インデックス番号を指定して値を取得して、結果を表示するだけのコードとなります。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
// Listを用意
var list = new List<int> { 1, 2, 3, 4, 5 };
try
{
Console.WriteLine(list.ElementAt(4));
}
catch (ArgumentOutOfRangeException e)
{
System.Console.WriteLine(e.ToString());
}
catch (Exception e)
{
System.Console.WriteLine(e.ToString());
}
}
}
}
実行結果

以下のように、INDEX番号が範囲にない場合は「ArgumentOutOfRangeException」が発生します。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
// Listを用意
var list = new List<int> { 1, 2, 3, 4, 5 };
try
{
Console.WriteLine(list.ElementAt(5));
}
catch (ArgumentOutOfRangeException e)
{
System.Console.WriteLine(e.ToString());
}
catch (Exception e)
{
System.Console.WriteLine(e.ToString());
}
}
}
}
実行結果

「ElementAtOrDefault」を使用すると、INDEX番号が範囲にない場合に型のデフォルト値を返してくれます。
using System;
using System.Collections.Generic;
using System.Linq;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
// Listを用意
var list = new List<int> { 1, 2, 3, 4, 5 };
try
{
Console.WriteLine(list.ElementAtOrDefault(5));
}
catch (ArgumentOutOfRangeException e)
{
System.Console.WriteLine(e.ToString());
}
catch (Exception e)
{
System.Console.WriteLine(e.ToString());
}
}
}
}
実行結果

-
前の記事
Vue.js クリックイベント時にENTERキーイベントも取得する 2022.02.23
-
次の記事
MySQL insert後のオートインクリメント値を確認する 2022.02.23
コメントを書く