C# プロパティを使用する
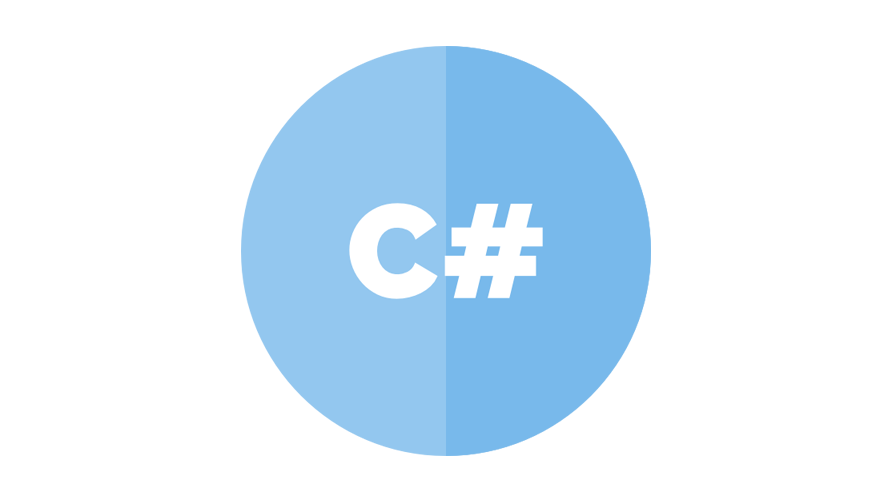
C#で、プロパティを使用するるサンプルコードを記述してます。
環境
- OS windows10 pro 64bit
- Microsoft Visual Studio Community 2019 Version 16.7.1
プロパティを使用
以下は、プロパティを使用して、人の名前と年齢を指定して、取得するコードとなります。
using System;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
Human human = new Human();
human.Name ="mebee";
human.Age = 15;
Console.WriteLine(" name : " + human.Name + " age : " + human.Age);
}
}
class Human
{
private string name;
private int age;
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
public int Age
{
get
{
return age;
}
set
{
age = value;
}
}
}
}
実行結果

以下のように、省略して記述することも可能です。
using System;
namespace testapp
{
class Program
{
static void Main(string[] args)
{
Human human = new Human();
human.Name ="mebee";
human.Age = 15;
Console.WriteLine(" name : " + human.Name + " age : " + human.Age);
}
}
class Human
{
public string Name { get; set; }
public int Age { get; set; }
}
}
-
前の記事
javascript イベント発生元のfileのサイズを取得する 2021.09.15
-
次の記事
AlmaLinux TeamViewerをインストールする 2021.09.15
コメントを書く