python pandasでDataFrameの列の合計値を求める
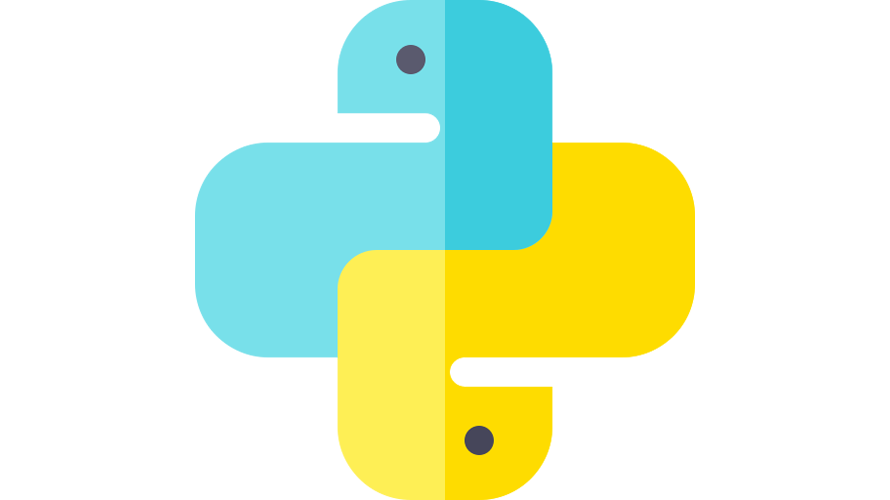
pythonで、ライブラリpandasのsumを使用して、DataFrameの列の合計値を求めるサンプルコードを記述してます。pythonのバージョンは3.8.5を使用してます。
環境
- OS windows10 pro 64bit
- python 3.8.5
pandasインストール
pandasをインストールされていない方は、pipでインストールしておきます。
pip install pandas
# numpyも使用するのでインストールしておきます
pip install numpy
sum使い方
sumを使用すると、DataFrameの列の合計値を求めることが可能です。
import pandas as pd
DataFrame['列名'].sum()
以下は、ランダムな値で生成した3行5列のDataFrameの列の合計値を求めるサンプルコードとなります。
import numpy as np
import pandas as pd
df = pd.DataFrame(
np.random.randint(1,10,size=(5, 3)),
columns=list('123'))
print(df)
# 1 2 3
# 0 1 8 6
# 1 1 3 4
# 2 1 7 3
# 3 6 4 5
# 4 2 8 9
sum = df['1'].sum()
print ("列1 合計:",sum)
# 列1 合計: 11
sum = df['2'].sum()
print ("列2 合計:",sum)
# 列2 合計: 30
sum = df['3'].sum()
print ("列3 合計:",sum)
# 列3 合計: 27
-
前の記事
Ruby 「三項演算子」と「if文」のパフォーマンスを計測する 2021.07.15
-
次の記事
Nuxt.js ライブラリ「vue-simple-search-dropdown」を使用してシンプルなドロップダウンリストを作成する 2021.07.15
コメントを書く