ASP.NET Core PostgreSQLと接続する
- 作成日 2021.06.03
- 更新日 2022.02.26
- ASP.NET Core PostgreSQL
- ASP.NET Core, PostgreSQL
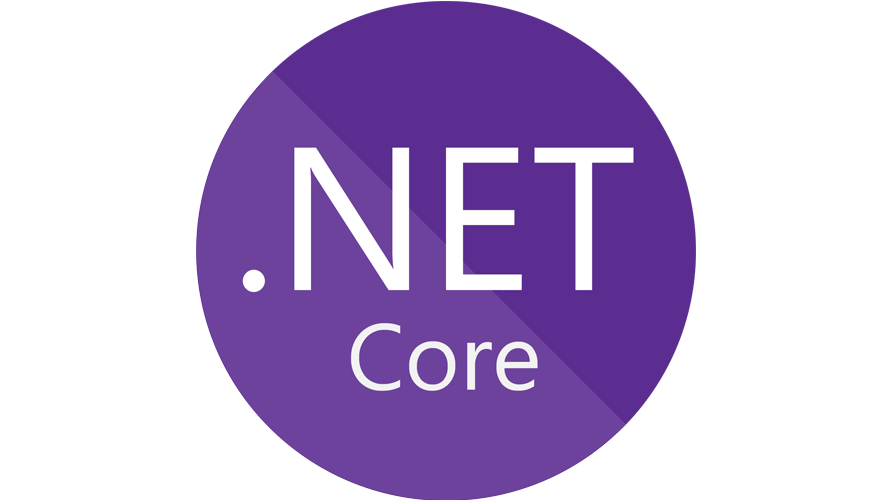
ASP.NET CoreでPostgreSQLと接続するまでの手順を記述してます。.NET Coreのバージョンは3.1を使用してます。
環境
- OS windows10 pro
- IDE Visual Studio 2019
- .NET Core 3.1
- PostgreSQL 13.1
プロジェクト作成
Visual Studio 2019からプロジェクトを作成します。
ASP.NET Core Webアプリケーションを選択します。
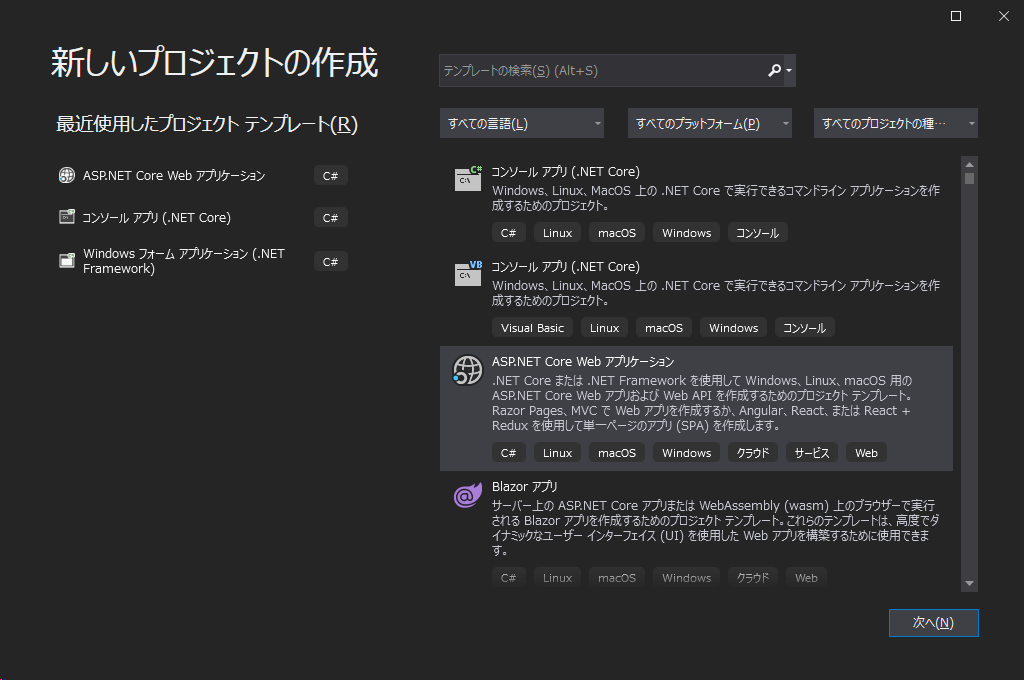
任意の名前でプロジェクトを作成します。
※ここでは「postgretest」として作成してます。
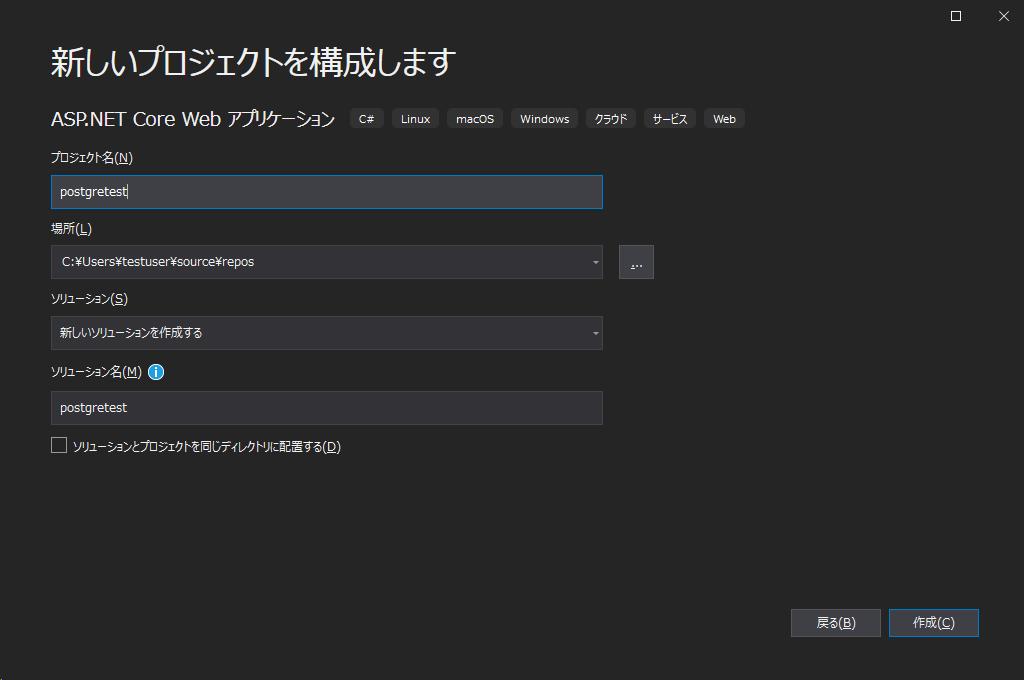
「空」を選択します。
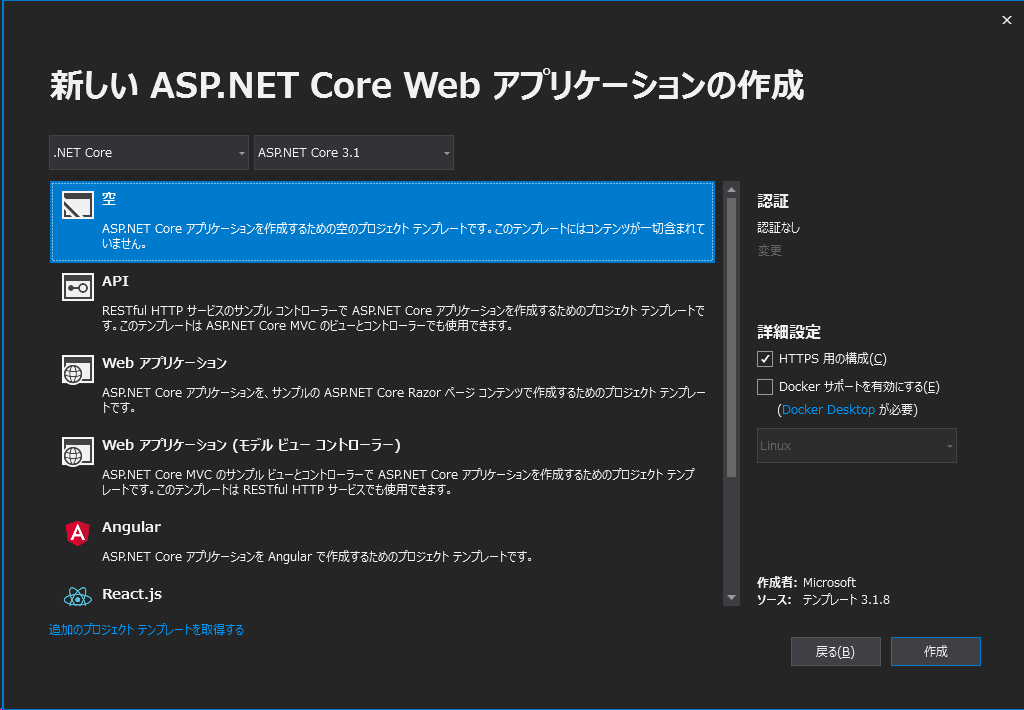
プロジェクトが作成されます。
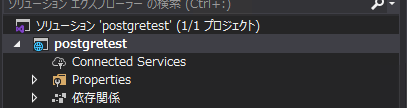
パッケージ追加
NuGetパッケージから以下の3つをインストールしておきます。
- Microsoft.EntityFrameworkCore.Tools
- Npgsql.EntityFrameworkCore.PostgreSQL
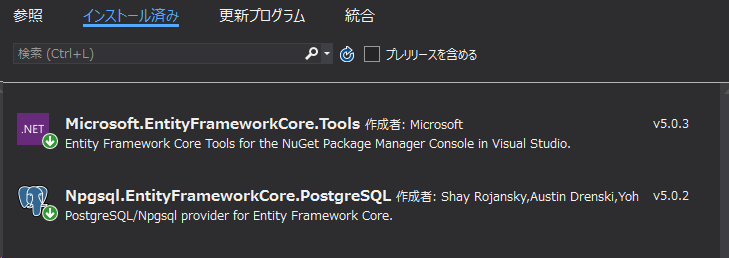
パッケージマネージャーから
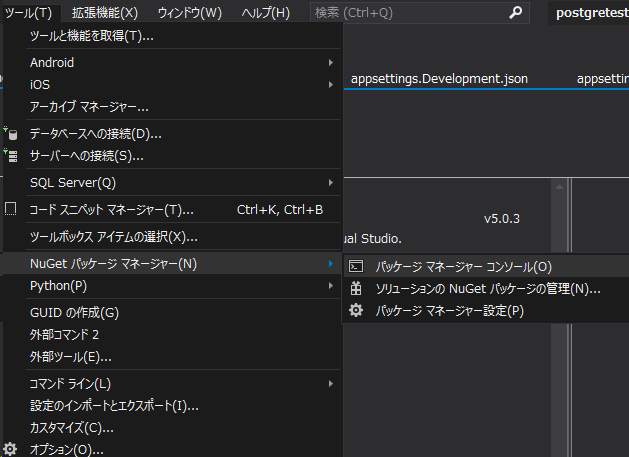
以下のコマンドで、インストール可能です。
Install-Package Microsoft.EntityFrameworkCore.Tools
Install-Package Npgsql.EntityFrameworkCore.PostgreSQL
DB作成
PostgreSQL側でDBを作成しておきます。
※ここでは「mydb」を作成しておきます。
create database mydb;
接続文字列追加
「appsettings.json」とデバック用の「appsettings.Development.json」にPostgreSQLとの接続文字列を追加しておきます。
サーバー localhost
DB名 mydb
ユーザー名 pgadmin
パスワード password
で追加してます。
"ConnectionStrings": {
"DefaultConnection": "Host=localhost;Database=mydb;Username=pgadmin;Password=password"
},
Modelsクラス作成
まずは、プロジェクト配下に「Models」フォルダを作成して、そこに「Customer.cs」を作成します。

「Customer.cs」を以下のように記述します。
※ここでは単純にカラム「ID」と「Name」があるテーブル「Customer」に「mydb」に追加します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace postgretest.Models
{
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
}
}
マッピング
マッピングを行うため、プロジェクト配下に「Data」フォルダを作成して、そこに「CustomerContext.cs」を作成します。

「CustomerContext.cs」を以下のように記述します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
using postgretest.Models;
namespace postgretest.Data
{
public class CustomerContext : DbContext
{
public CustomerContext(DbContextOptions<CustomerContext> options) : base(options)
{
}
public DbSet<Customer> Customer { get; set; }
}
}
サービス登録
「Startup.cs」にサービスを登録します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Configuration;
using postgretest.Data;
namespace postgretest
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<CustomerContext>(options =>
{
options.UseNpgsql(Configuration.GetConnectionString("DefaultConnection"));
});
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapGet("/", async context =>
{
await context.Response.WriteAsync("Hello World!");
});
});
}
}
}
Migration実行
パッケージマネージャーコンソールを開いて、Migrationを実行します。
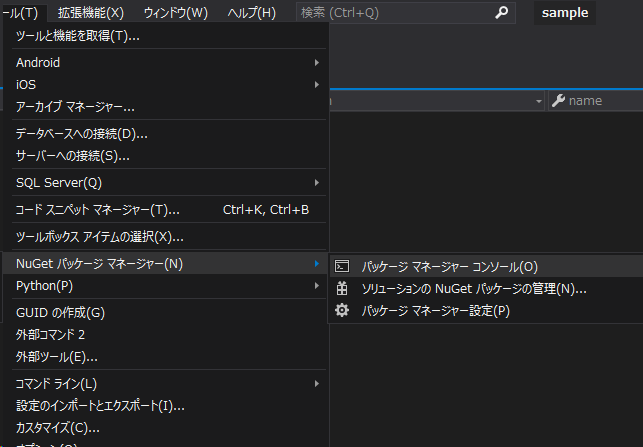
以下のコマンドを実行します。
Add-Migration Initial
「Migrations」フォルダ配下にマイグレーションファイルが作成されます。

テーブルを作成します。
Update-Database
テーブルが作成されていることが確認できます。
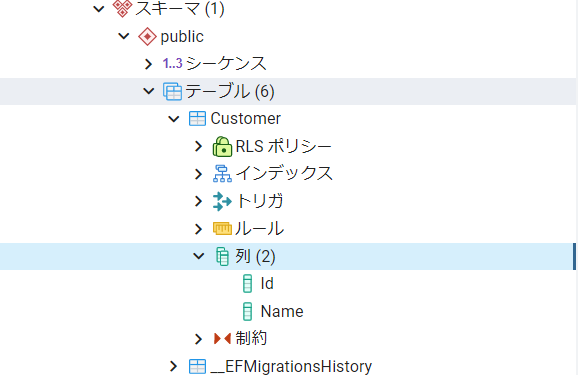
-
前の記事
windows プロダクトキーを確認する方法 2021.06.03
-
次の記事
.NET Coreを3.0から3.1にアップデートする 2021.06.03
コメントを書く