google drive apiで指定したフォルダ内のファイルを抽出する
- 作成日 2023.12.02
- Google Drive
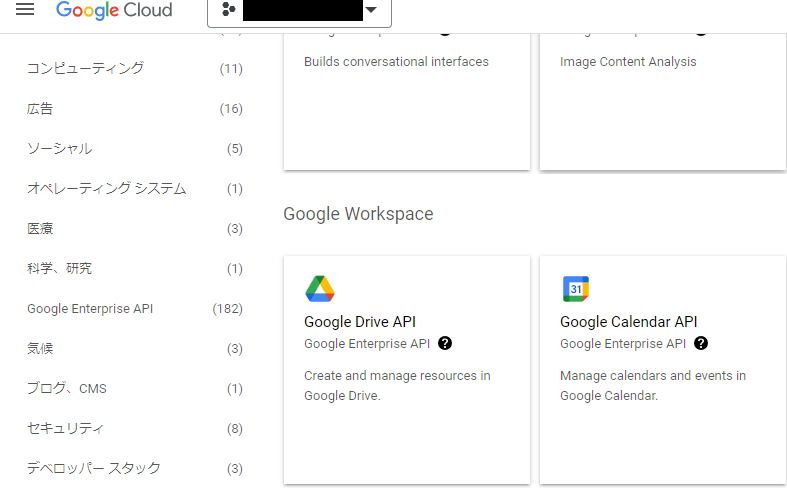
google drive apiを使用して、指定したIDのフォルダ内のファイルを抽出するサンプルコードを記述してます。ここではpythonを使用してます。
環境
- OS windows11 home
- python のバージョンは3.10.0です。
事前準備
google cloudにアクセスして、google drive apiを有効しておきます。
Google Drive APIを選択します。
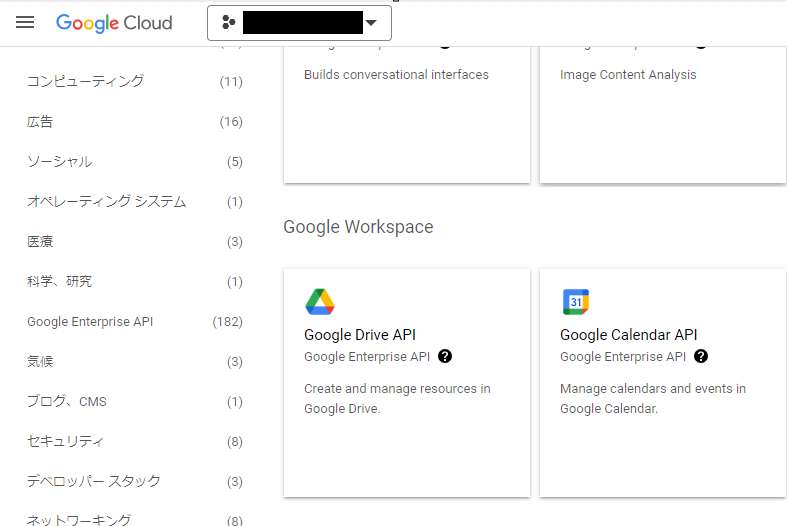
「有効にする」ボタンをクリックして有効しておきます。
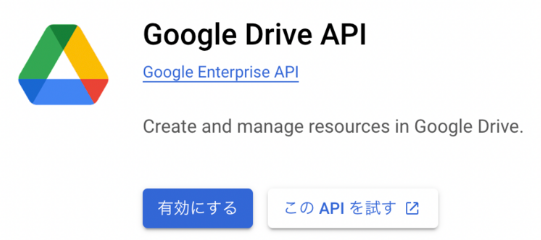
次に「認証情報を作成」から「OAuth」の設定を行います。
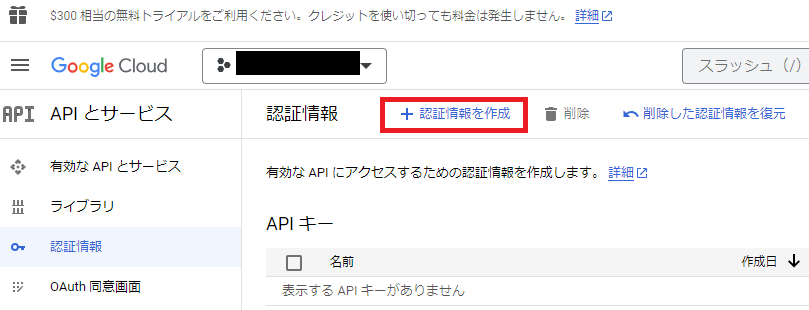
「OAuth クライアントID」を選択します。
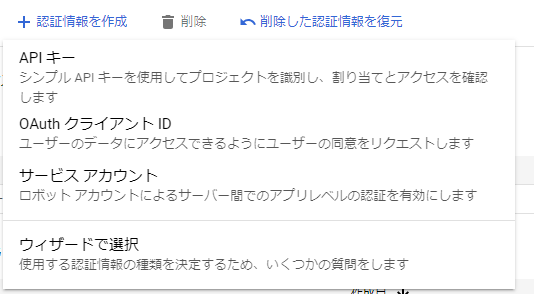
ここでは「デスクトップアプリ」を選択して「作成」ボタンを押下して作成します。
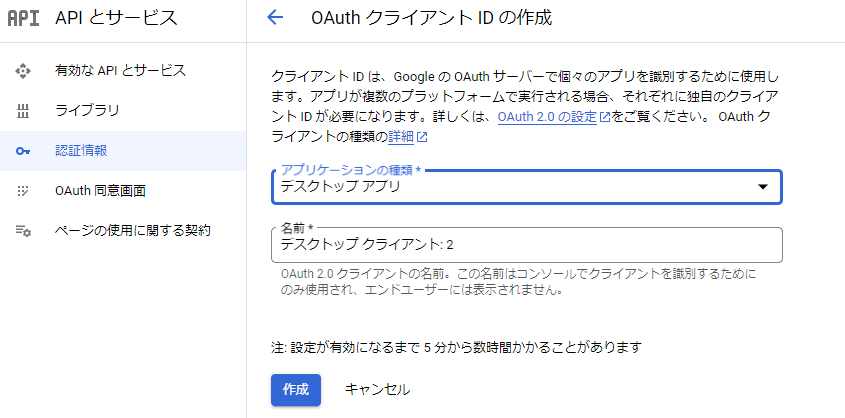
作成した後は、「JSON」をダウンロードしておきます。後でプログラムで使用します。
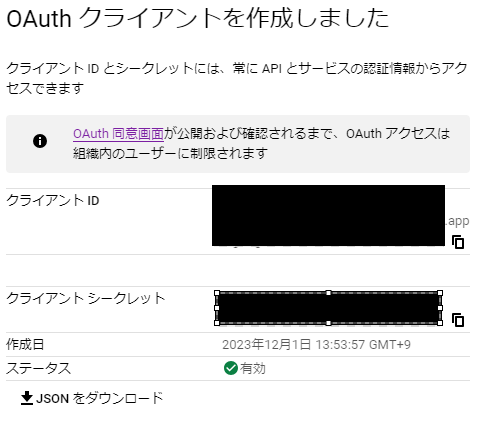
ライブラリのインストール
事前に必要なライブラリを、pipでインストールしておきます。
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib oauth2client
プログラム作成
以下のコードを実行します。
※さきほどダウンロードしたjsonを「client_secret.json」にリネームして、コード同じ階層において下さい。
import os
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
# Google Drive APIのスコープ
SCOPES = ['https://www.googleapis.com/auth/drive']
def authenticate():
creds = None
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json')
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'client_secret.json', SCOPES)
creds = flow.run_local_server(port=0)
with open('token.json', 'w') as token:
token.write(creds.to_json())
return creds
def get_folder_files(folder_id):
creds = authenticate()
service = build('drive', 'v3', credentials=creds)
# フォルダ内のファイル情報を取得
results = service.files().list(q=f"'{folder_id}' in parents",
fields="files(id, name, mimeType)").execute()
files = results.get('files', [])
if not files:
print('No files found.')
else:
print('Files:')
for file in files:
print(f"Name: {file['name']}, ID: {file['id']}, Type: {file['mimeType']}")
if __name__ == '__main__':
folder_id = '検索したいフォルダのIDを指定'
get_folder_files(folder_id)
実行するとブラウザ条で、アカウントの選択画面が表示されます。
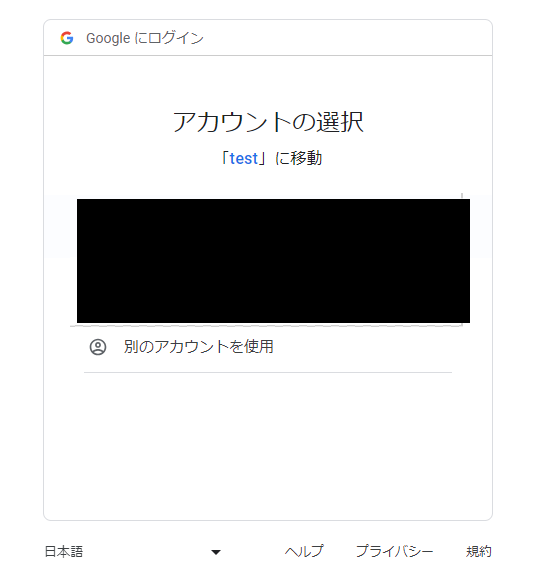
許可をクリックして下さい。
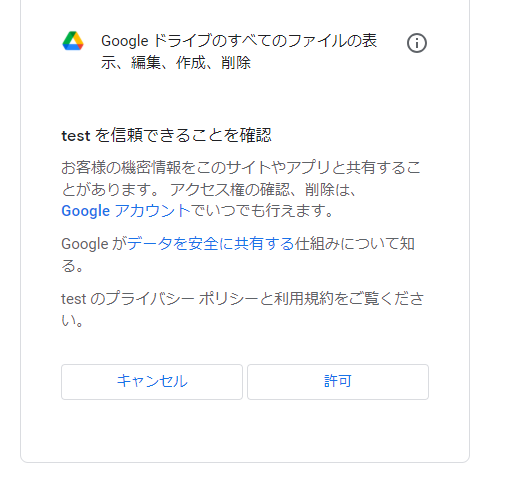
以下のメッセージが表示されると許可が完了します。


再度、プログラムを実行で指定したフォルダ内のファイルが一覧で取得されます。
-
前の記事
kotlin Listの要素を頭に文字を付けて全て連結させる 2023.12.02
-
次の記事
Android Studio コードを折りたたむショートカットキー 2023.12.02
コメントを書く